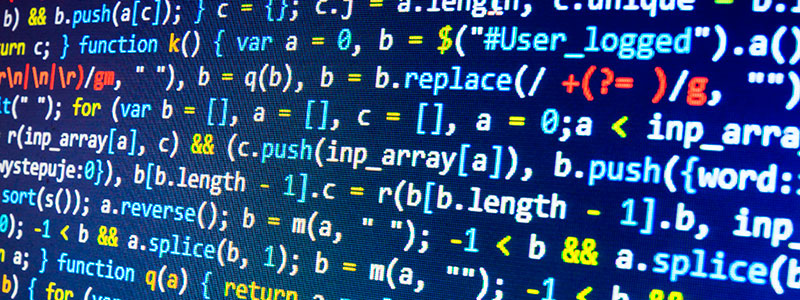

-
Using comments as arguments in python.
Python allows programmers to pass additional arguments to functions via comments. Now armed with this knowledge head out and spread it to all code bases.
Feel free to use the code I wrote in your projects.
Link to the source code: https://github.com/raldone01/python_lessons_py/blob/v2.0.0/lesson_0_comments.ipynb
Image transcription:
```python
First we have to import comment_arguments from arglib
Sadly arglib is not yet a standard library.
from arglib import comment_arguments
def add(*args, **kwargs): c_args, c_kwargs = comment_arguments() return sum([int(i) for i in args + c_args])
Go ahead and change the comments.
See how they are used as arguments.
result = add() # 1, 2 print(result)
comment arguments can be combined with normal function arguments
result = add(1, 2) # 3, 4 print(result) ```
---
Output:
3 10
This is version
v2.0.0
of the post: https://github.com/raldone01/python_lessons_py/tree/v2.0.0Note:
v1.0.0
of the post can be found here: https://github.com/raldone01/python_lessons_py/tree/v1.0.0Choosing
lib
as the name for my module was a bit devious. I did it because I thought if I am creating something cursed why not go all the way?Regarding misinformation:
I thought simply posting this in programmer humor was enough. Anyways, the techniques shown here are not yet regarded best practice. Decide carefully if you want to apply the shown concepts in your own code bases.
-
My issue with every chatbot I've tried
That's not universal. For instance, last week I got help writing a bash script. But I hope they're helping lots of you in lots of ways.
-
shell-mommy is a program that encourages users while using command line applications.
github.com GitHub - sudofox/shell-mommy: Mommy is here for you on the command line ~ ❤️Mommy is here for you on the command line ~ ❤️. Contribute to sudofox/shell-mommy development by creating an account on GitHub.
-
Every OS Sucks - a song from circa 2001 by Three Dead Trolls in a Baggie
YouTube Video
Click to view this content.
https://en.wikipedia.org/wiki/Three_Dead_Trolls_in_a_Baggie
I gave up looking for the year this was actually written but it existed on mp3.com in 2001.
-
Real Programmers Implement Functions On Top Of Classes
```js class BaseFunction { static #allowInstantiation = false;
constructor(...args) { if (!BaseFunction.#allowInstantiation) { throw new Error( "Why are you trying to use 'new'? Classes are so 2015! Use our fancy 'run' method instead!" ); } for (const [name, validator] of this.parameters()) { this[name] = validator(args.shift()); } }
parameters() { return []; }
body() { return undefined; }
static run(...args) { BaseFunction.#allowInstantiation = true; const instance = new this(...args); BaseFunction.#allowInstantiation = false; return instance.body(); } }
class Add extends BaseFunction { parameters() { return [ ["a", (x) => Number(x)], ["b", (x) => Number(x)], ]; }
body() { return this.a + this.b; } }
console.log(Add.run(5, 3)); // 8
```